Automating URL Submission to Bing Using IndexNow and Google Apps Script
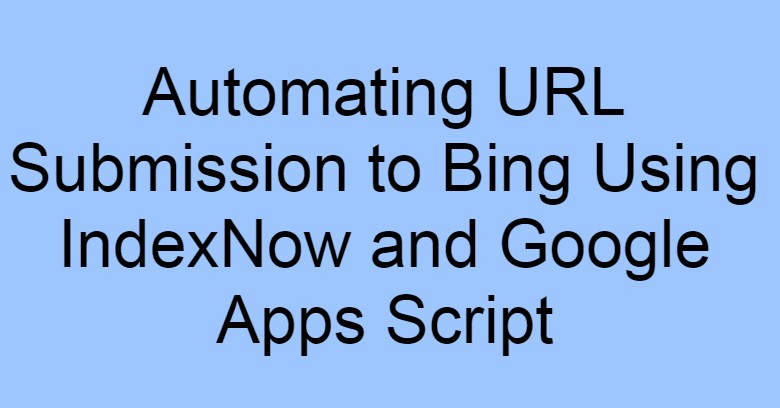
Automating URL Submission to Bing Using IndexNow and Google Apps Script
In this guide, we will demonstrate how to automate URL submission to Bing using the IndexNow API. We will also show how to fetch URLs directly from an RSS feed using Google Apps Script and store them in Google Sheets.
Table of Contents
- Why Use IndexNow?
- Steps to Automate URL Submission
- Example Google Apps Script Code
- Fetching RSS Feed Data
- Conclusion
Why Use IndexNow?
IndexNow is a powerful and easy-to-use API that instantly notifies search engines about changes to your website. This ensures that your new or updated content is indexed promptly. Using Google Apps Script, you can automate this process and regularly submit URLs from your Google Sheets document.
Steps to Automate URL Submission
The following steps outline how to automate the URL submission process to Bing using Google Apps Script:
- Retrieve URLs from the RSS Google Sheets page.
- Check the Sent page to avoid duplicate submissions.
- Submit the URLs in batches to Bing’s IndexNow API.
- Log the responses for future reference.
Example Google Apps Script Code
The following script is designed to automatically submit URLs from a Google Sheets document to Bing’s IndexNow API:
// Function to submit URLs to Bing IndexNow API
function submitUrlBatch() {
var apiKey = 'YOUR_API_KEY';
var siteUrl = 'YOUR_SITE_URL';
var rssSheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName('RSS');
var sentSheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName('Sent');
if (!sentSheet) {
sentSheet = SpreadsheetApp.getActiveSpreadsheet().insertSheet('Sent');
}
var sentUrls = sentSheet.getRange('A1:A' + sentSheet.getLastRow()).getValues().flat();
var urls = rssSheet.getRange('B2:B').getValues();
var urlList = [];
var sendCount = 0;
// Check if URLs are already sent
for (var i = 0; i < urls.length; i++) {
var url = urls[i][0];
if (url && sentUrls.indexOf(url) === -1) {
urlList.push(url);
var lastRow = sentSheet.getLastRow();
sentSheet.getRange(lastRow + 1, 1).setValue(url); // Save URL in 'Sent' sheet
sendCount++;
}
}
if (sendCount > 0) {
// Create payload for IndexNow API
var payload = JSON.stringify({
host: siteUrl,
key: apiKey,
keyLocation: siteUrl + '/key.txt',
urlList: urlList
});
// Set options for the HTTP POST request
var options = {
'method': 'POST',
'contentType': 'application/json',
'payload': payload
};
// Send the payload to Bing IndexNow API
var response = UrlFetchApp.fetch('https://api.indexnow.org/indexnow', options);
Logger.log(response.getResponseCode()); // Log response code
Logger.log(response.getContentText()); // Log response content
} else {
Logger.log('No new URLs to submit.');
}
}
Fetching RSS Feed Data
You can automate the process of fetching URLs from an RSS feed using Google Apps Script. The following script pulls titles and URLs from a given RSS feed and stores them in a Google Sheets document:
// Function to fetch RSS feed and save to Google Sheets
function fetchRSSFeed() {
var sheetName = 'RSS';
var spreadsheet = SpreadsheetApp.getActiveSpreadsheet();
var sheet = spreadsheet.getSheetByName(sheetName);
// If "RSS" sheet doesn't exist, create it
if (!sheet) {
sheet = spreadsheet.insertSheet(sheetName);
}
var rssUrl = 'YOUR_RSS_FEED_URL';
// Fetch the RSS feed
var response = UrlFetchApp.fetch(rssUrl);
var xml = response.getContentText();
// Parse the XML
var document = XmlService.parse(xml);
var root = document.getRootElement();
// Define namespace
var atomNS = XmlService.getNamespace('http://www.w3.org/2005/Atom');
// Get "entry" elements
var entries = root.getChildren('entry', atomNS);
// Clear the sheet
sheet.clear();
// Add headers
sheet.getRange(1, 1).setValue('Title');
sheet.getRange(1, 2).setValue('URL');
// Loop through entries and write titles and URLs to the sheet
for (var i = 0; i < entries.length; i++) {
var entry = entries[i];
// Get the title
var title = entry.getChild('title', atomNS).getText();
// Find the link with rel='alternate'
var links = entry.getChildren('link', atomNS);
var linkUrl = '';
for (var j = 0; j < links.length; j++) {
var link = links[j];
if (link.getAttribute('rel').getValue() == 'alternate') {
linkUrl = link.getAttribute('href').getValue();
break;
}
}
// Write title and URL to the sheet
sheet.getRange(i + 2, 1).setValue(title);
sheet.getRange(i + 2, 2).setValue(linkUrl);
}
Logger.log('RSS feed processed successfully.');
}
Conclusion
By automating the URL submission process to Bing's IndexNow API and fetching URLs from your RSS feed, you can ensure that your website is indexed more quickly and efficiently. The above Google Apps Scripts help you manage your URL submissions and fetch the latest content directly from your RSS feed into Google Sheets.
Comments
Post a Comment